Mastering Ruby on Rails: Unescape HTML for Seamless Web Development
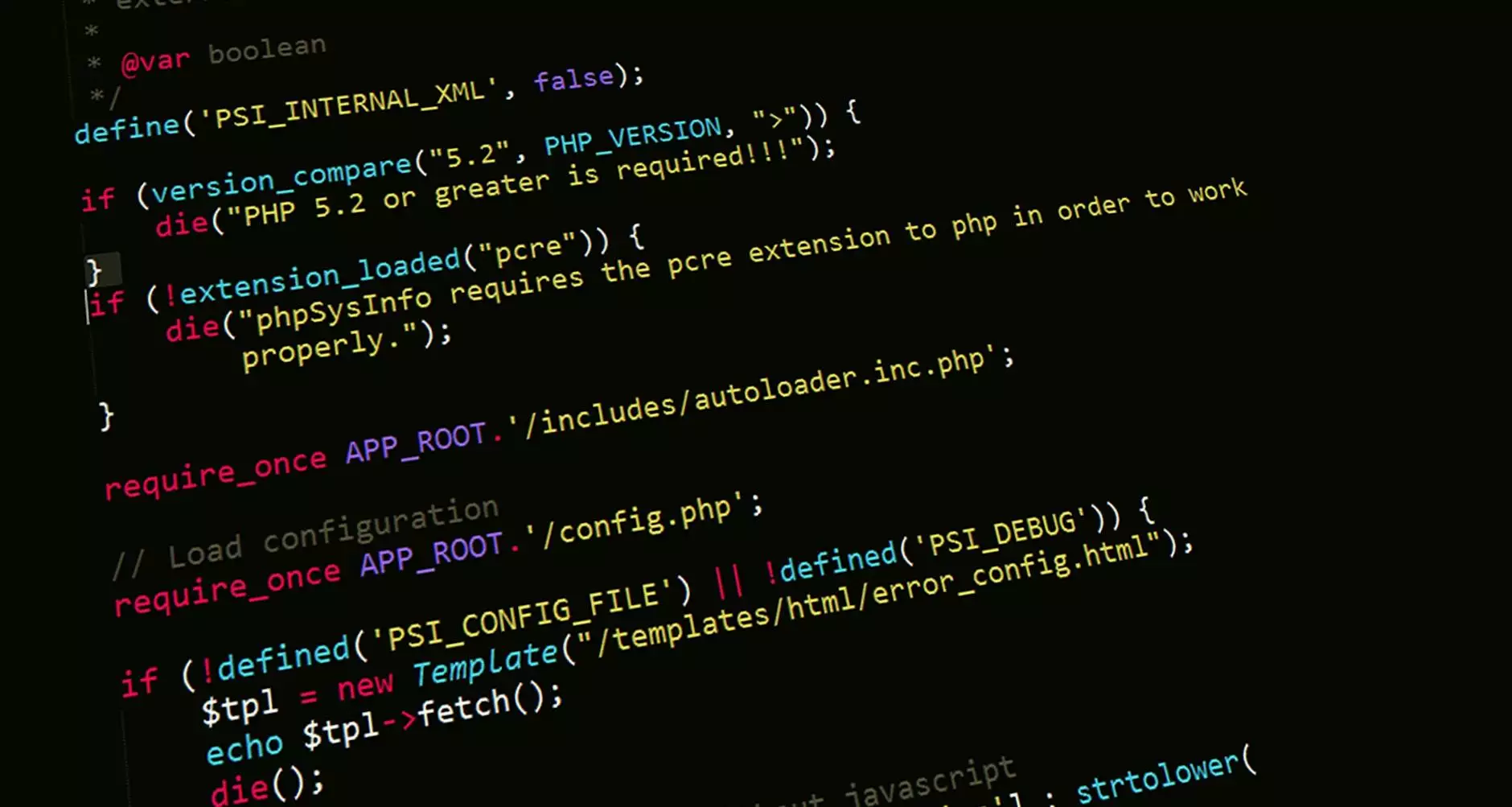
In the ever-evolving realm of web development, Ruby on Rails has established itself as a premier framework, empowering developers to create dynamic, high-performance applications with remarkable efficiency. One key aspect of web development is handling HTML content properly. This article dives deep into the essential topic of how to unescape HTML in Ruby on Rails, a crucial skill for web developers aiming to maintain clean and efficient codebases. The keyword ruby on rails unescape html 0789225888 will be integral as we explore practical techniques and beneficial insights.
Understanding HTML Escape and Unescape
Before we delve into the specifics of unescaping HTML in Ruby on Rails, it’s vital to understand what HTML escaping and unescaping actually mean. HTML escaping is the process of converting special characters in HTML (such as &, , etc.) into their corresponding HTML entities (&, , ...). This step is critical for preventing Cross-Site Scripting (XSS) attacks and other vulnerabilities that can compromise web applications. However, in certain contexts, you may need to retrieve the original content from those escaped characters - hence the need to unescape HTML.
Importance of Unescaping HTML in Rails Applications
Unescaping HTML is not just a technical necessity but a vital part of improving user experience on web applications. When restoring data that has been stored in an escaped state, developers need to ensure that content displays as intended without compromising security. This process is particularly significant for applications that handle user-generated content.
Why Use Ruby on Rails for HTML Management?
Ruby on Rails simplifies many aspects of web development, including HTML management. With built-in helpers and tools, developers can effortlessly escape and unescape HTML content. Below are some reasons why Ruby on Rails is an optimal choice for handling HTML:
- Simplicity: Ruby on Rails has a clean and readable syntax that makes managing HTML content intuitive.
- Security: The framework offers robust mechanisms to prevent common security threats.
- Community Support: An extensive community means numerous plugins, gems, and resources are available for developers.
- Efficiency: Rails fosters rapid development through conventions that save time and reduce boilerplate code.
Techniques to Unescape HTML in Ruby on Rails
Using Built-in Rails Functions
Ruby on Rails provides several built-in methods for unescaping HTML. The most common methods include:
- html_safe: This method marks a string as safe for HTML output.
- CGI::unescapeHTML: This method from the CGI module can convert escaped characters back to their original characters.
Example of Unescaping with CGI Module
Here’s a simple example demonstrating how you can use the CGI module to unescape HTML:
require 'cgi' escaped_string = "Hello & Welcome to " unescaped_string = CGI.unescapeHTML(escaped_string) puts unescaped_string # Outputs: Hello & Welcome toCreating a Custom Helper Method
In addition to using built-in methods, you may want to create a custom helper for unescaping HTML. Here’s how you can do it:
module ApplicationHelper def unescape_html(text) CGI.unescapeHTML(text) end endThis custom helper allows you to easily unescape HTML from anywhere in your application. Simply call unescape_html with the desired string as its argument.
Best Practices for Unescaping HTML
While unescaping HTML is essential, it’s crucial to follow best practices to avoid introducing vulnerabilities:
- Always Validate Inputs: Ensure any user-generated content is validated before processing to mitigate XSS risks.
- Use Safe HTML Libraries: Rely on established libraries that handle HTML safely and effectively.
- Limit Unescaped Output: Only unescape HTML when absolutely necessary and keep track of where it’s used within your application.
Case Study: Implementing Unescaped HTML in a Travel Blog
Consider a travel blog like The Broad Life. Managing content dynamically—such as user comments or articles—often requires unescaping HTML. Here’s a practical example of how unescaped HTML can enhance user experience on a travel blog:
User Comments Section
When users leave comments on blog posts, these comments will often include formatting characters (like , ) that need to be displayed correctly. Using unescape functionality ensures these characters appear as intended:
User:
Conclusion: The Power of Proper HTML Management
In conclusion, effectively handling HTML content is a cornerstone of modern web development. Using techniques provided by Ruby on Rails to unescape HTML, developers can create a robust and secure user experience. As you develop your applications, remember the importance of validating inputs and maintaining security.
By mastering these techniques, you will not only improve your coding skills but also enhance the value of your web applications, making them safer and more user-friendly. Whether you’re developing a personal blog or a complex business platform, the skills you gain in unescaping HTML will serve you well throughout your development career.
Further Reading and Resources
If you are eager to learn more about Ruby on Rails and HTML management, consider exploring the following resources:
- Rails Official Website
- Ruby on Rails Guides
- Ruby Quick Start
- Pluralsight - Ruby on Rails Course
By leveraging these tools and insights, you can ensure your Ruby on Rails applications are top-notch, paving the way towards a successful web development journey.